Unlocking Python CLI Capabilities Without Writing Code
Written on
Chapter 1: Introduction to Python CLI Tools
Python's standard library comes packed with an array of useful modules that offer command line interfaces (CLI). These tools enable you to accomplish a variety of tasks directly from the terminal without the need to open any Python files. From starting a web server to parsing JSON, these capabilities will be explored throughout this article.
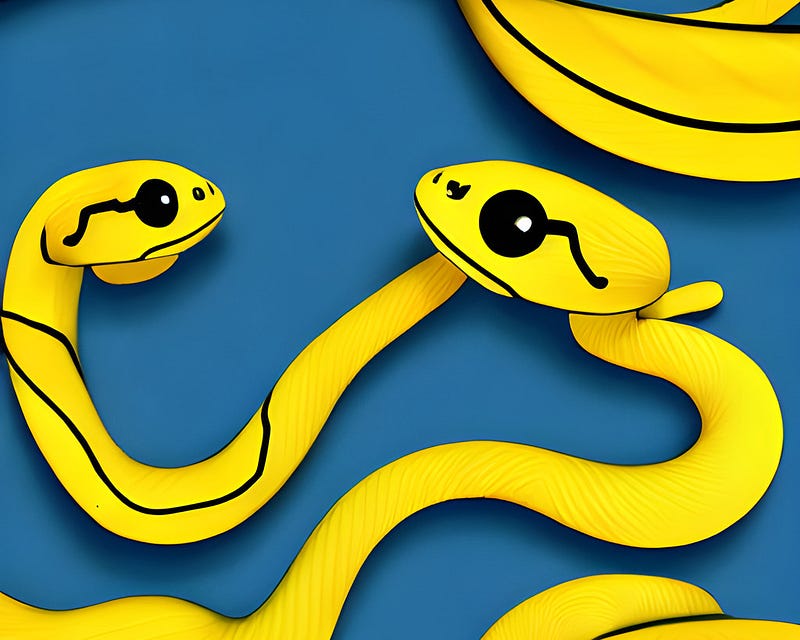
Section 1.1: Benchmarking with Timeit
One of the most commonly used Python modules for command line benchmarking is timeit. This tool allows for straightforward performance testing:
# Example usage
python -m timeit -s "import math" "math.sqrt(2)"
If your testing requires some setup, the -s option is invaluable for creating variables or importing modules, and it can be used multiple times. The number of loops can vary significantly; in one instance, you might execute 50 million loops, while in another, just 2,000. To enforce a specific loop count, use the -n X option. The output indicates the best of five runs by default, but this can be adjusted using the -r X option.
For a more robust benchmarking experience, consider using pyperf, which provides a familiar interface to timeit, yet with enhanced features:
# Example usage
python -m pyperf timeit --repeat=5 --loops=2000 "math.sqrt(2)"
pyperf generates detailed statistics that can be saved and analyzed further, making it a great choice for performance comparisons across different Python versions.
Section 1.2: Running Simple HTTP Servers
If you require a simple HTTP server for testing purposes, Python's http.server module makes it incredibly easy to set one up:
# Command to run HTTP server
python -m http.server
While this is perfect for testing, it's not suitable for production environments. For more robust applications, consider employing a WSGI server like Gunicorn.
If you need a full-featured FTP server, the pyftpdlib library can fulfill that need:
# Example usage for FTP server
from pyftpdlib.authorizers import DummyAuthorizer
from pyftpdlib.handlers import FTPHandler
from pyftpdlib.servers import FTPServer
authorizer = DummyAuthorizer()
authorizer.add_user("user", "12345", "/home/user", perm="elradfmwMT")
handler = FTPHandler
handler.authorizer = authorizer
server = FTPServer(("0.0.0.0", 21), handler)
server.serve_forever()
Chapter 2: Debugging and Profiling
The first video titled "Build AWESOME CLIs With Click in Python" offers insights into creating command line interfaces using Python's Click library.
For debugging, while IDEs are convenient, Python’s pdb module allows you to debug directly from the terminal:
# Running a script with pdb
python -m pdb your_script.py
This command launches the debugger and allows you to set breakpoints, inspect variables, and step through code. If you encounter obscure errors, the faulthandler module can help reveal more context by dumping tracebacks.
Finally, for profiling Python applications, cProfile provides useful insights into function call performance:
# Profiling a script
python -m cProfile your_script.py
For a comprehensive understanding of profiling, refer to my previous article on the topic.
Section 2.1: JSON Parsing from CLI
Python’s standard library includes a json module that also offers a command line interface. You can validate and format JSON directly in the terminal:
# Example command for JSON validation
echo '{"key": "value"}' | python -m json.tool
This is especially beneficial in environments lacking advanced JSON tools.
Section 2.2: Compression Techniques
The standard library provides various modules for file compression and decompression, such as gzip and zipfile. For instance:
# Compressing files with gzip
python -m gzip myfile.txt
To create a ZIP archive from a directory, the zipfile module is appropriate:
# Creating a ZIP archive
python -m zipfile -c archive.zip my_directory/*
Chapter 3: Browser Automation
The second video titled "Python (Fall 2024) Module 4-10: Command Line UI Tricks" delves into various command line tricks and techniques in Python.
Among the lesser-known modules in Python’s standard library is webbrowser, which can open a web browser directly from the command line:
# Opening a web browser
python -m webbrowser -t "http://www.example.com"
Closing Remarks
Python excels in providing various command line tools for everyday tasks, most of which are integrated into its standard library. Exploring these resources can greatly enhance your productivity and streamline workflows. I encourage you to peruse the documentation to uncover even more useful features.
This article was originally published at martinheinz.dev, where you can read further insights and tips on Python programming.