How to Verify Background Image Loading with JavaScript
Written on
Chapter 1: Introduction to Background Image Loading
In web development, it's often necessary to verify if a background image has been successfully loaded using JavaScript. This guide will explore how to perform this check effectively.
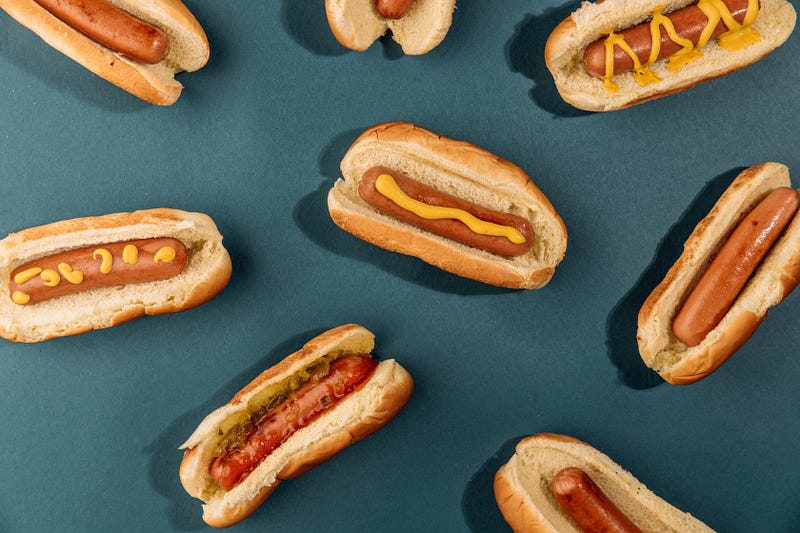
Photo by Ball Park Brand on Unsplash
Sometimes, developers need to ensure that a background image is fully loaded before applying it to a webpage. In this article, we will demonstrate how to detect if a background image has loaded using JavaScript.
Section 1.1: Listening for the Load Event
To check if a background image has loaded, we can listen for the load event associated with the image. When the image is fully loaded, we can then apply it as the background image.
For example, consider the following code snippet:
const bgElement = document.querySelector("body");
let preloaderImg = document.createElement("img");
preloaderImg.src = imageUrl;
preloaderImg.addEventListener('load', (event) => {
bgElement.style.backgroundImage = url(${imageUrl});
preloaderImg = null;
});
In this example, we define the imageUrl variable with the URL of the image we want to use as the background. We use document.querySelector to select the body element, and then create an img element to preload the specified image.
We set the src attribute of the preloaderImg to our imageUrl. By adding an event listener for the 'load' event on preloaderImg, we ensure that the callback function executes once the image has fully loaded.
Once it has loaded, we set the bgElement.style.backgroundImage to the loaded image. Finally, we set preloaderImg to null, effectively clearing the reference to the preloaded image.
Now, you should see the background image displayed on your webpage.
Subsection 1.1.1: Practical Implementation
In this section, we will watch a video that illustrates this concept in action.
The first video, "How To Load Images Like A Pro," provides practical insights into loading images efficiently.
Section 1.2: Conclusion
In summary, by listening for the load event of an image element, we can determine when an image has been successfully loaded. Once the image is ready, we can utilize its URL to set it as the background image of our page.
For additional resources, be sure to visit PlainEnglish.io. You can also subscribe to our free weekly newsletter, follow us on Twitter and LinkedIn, and join our Community Discord to connect with fellow developers.
Chapter 2: Advanced Background Image Techniques
Next, we will explore further techniques in handling background images with HTML and CSS.
The second video, "Background images with HTML & CSS," delves into the nuances of effectively managing background images in web development.