Unlocking Cleaner Code with Flutter Extensions: A Guide
Written on
Chapter 1: Introduction to Flutter Extensions
Flutter's elegant and expressive design has established it as a preferred framework for developing cross-platform mobile applications. As applications grow in complexity, the demand for cleaner, maintainable, and comprehensible code becomes critical. This is precisely where Dart extensions come into play. Extensions empower developers to augment existing classes with new functionalities without modifying the original code, making them essential for crafting more modular and readable code.
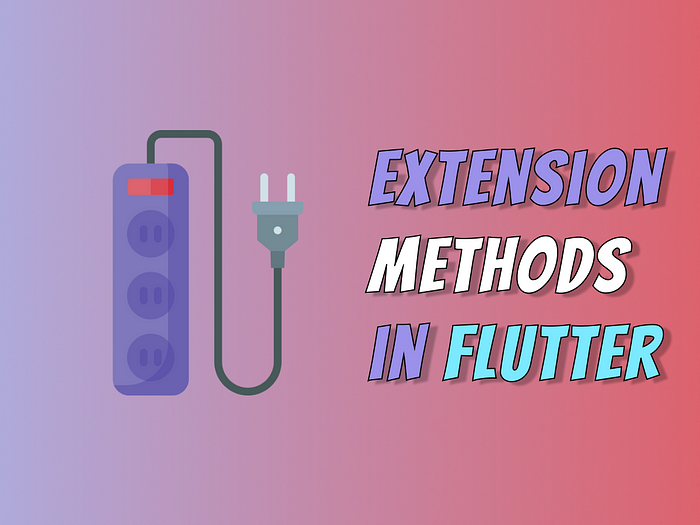
What Are Extensions?
Dart extensions allow the addition of methods and properties to any type, including built-in types from the Dart SDK or third-party libraries, without the need for subclassing. This capability is invaluable for enhancing classes with extra functionality seamlessly, leading to more concise, readable, and expressive code.
Making Code Cleaner and More Readable
A key benefit of using extensions is the ability to create cleaner and more intelligible code. Let’s look at a basic example involving a double extension.
Suppose you want to display a double value in a text widget as currency. Instead of appending the currency symbol repeatedly, you can define an extension.
extension DoubleExtension on double {
String get inr => "$this ₹";
String get usd => "$this $";
}
Now, you can use this extension in your text widgets as follows:
Text(100.00.inr) // INR
Text(100.00.usd) // USD
Notice how much more streamlined and maintainable the code has become—this illustrates the power of Dart extensions.
Chapter 2: Exploring Useful Extensions
In Flutter, extensions on BuildContext can significantly ease daily tasks. Let’s explore some of these extensions.
Flutter Clean Architecture Full Course for Beginners
This course covers essential concepts for beginners, including Bloc, Supabase, and Hive. It provides a comprehensive foundation for building scalable applications using Flutter.
Theme and Color Extensions
Prior to using extensions, accessing theme data or color schemes involved repetitive method calls:
final theme = Theme.of(context);
final primaryColor = theme.colorScheme.primary;
Using extensions simplifies this:
extension ThemeExtension on BuildContext {
ThemeData get theme => Theme.of(this);
}
extension ColorExtension on BuildContext {
ColorScheme get colorScheme => Theme.of(this).colorScheme;
Color get primary => colorScheme.primary;
}
You can then access properties directly, resulting in cleaner code:
final theme = context.theme;
final primaryColor = context.primary;
TextStyle Extensions
Before extensions, working with text styles could lead to verbose code. With extensions, you can streamline this:
extension TextStyleHelper on BuildContext {
TextStyle? get bodyMedium => Theme.of(this).textTheme.bodyMedium;
}
Now, applying styles becomes more readable:
Text("100.00", style: context.bodyMedium!.onPrimary(context).bold);
Conclusion
Extensions in Flutter are powerful tools that help developers write cleaner, more maintainable, and more understandable code. By leveraging extensions, you can encapsulate common functionalities, reduce boilerplate code, and enhance the modularity of your codebase. Whether working with localization, theming, navigation, or text styling, extensions can streamline and improve the efficiency of your Flutter applications.
So, whenever you encounter repetitive code or seek a more elegant approach to feature implementation, consider utilizing extensions—they might just be the solution you need.
I hope you found this guide valuable! If you enjoyed the information, feel free to support me by buying me a coffee! Your kindness would be greatly appreciated!
Follow Me