Understanding Key Differences Between astype() and to_datetime() in Pandas
Written on
Chapter 1: Introduction
Choosing the right method for data type conversion is crucial for efficient data analysis. In this guide, we will delve into the distinctions between the pandas.Series.astype() and pandas.to_datetime() methods for converting date-time strings to the datetime64[ns] data type. Both methods yield the same end result, but their performance and error handling vary significantly.
In previous articles, I've discussed useful strategies for handling date-time or time-series data in Python and Pandas. Let's examine the following key areas of focus:
- Performance Comparison of astype() and to_datetime()
- Date and Time Handling
- Error Management
Let’s get started!
Section 1.1: Performance Comparison
When comparing programming functions, evaluating their efficiency is vital. The performance of a method determines how quickly it processes data, especially when dealing with large datasets.
To gauge performance, we often look at execution time. A method that completes in less time is deemed more efficient. Let's illustrate this with an example by loading a sample sales dataset into a DataFrame.
import pandas as pd
df = pd.read_csv("Dummy_dates_sales.csv")
df.head()
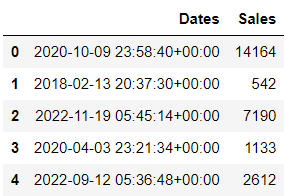
This dataset is a simple 100 x 2 table with a date-time column named "Dates" and an integer column "Sales." You can access this dataset for free from my GitHub repository.
Since Pandas recognizes the values in the "Dates" column as strings, we need to convert them into a date-time format. You can utilize the magic function %%timeit in Jupyter Notebook to measure execution time for both methods as follows:
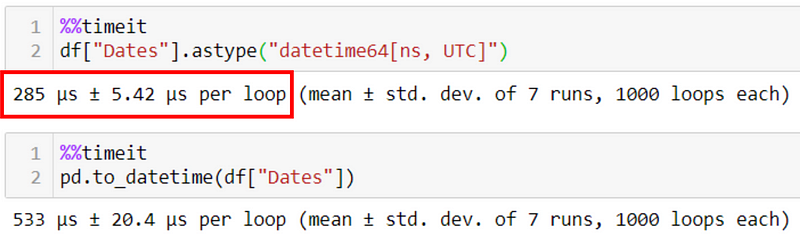
As shown, %%timeit executes the code 1000 times and averages the execution time for both astype() and to_datetime(). The results indicate that pandas.Series.astype() is approximately 1.87 times faster than to_datetime(), highlighting its efficiency.
Furthermore, astype() can convert multiple columns simultaneously, which to_datetime() cannot do.
Section 1.2: Date and Time Handling
While astype() can convert data types, it's not specifically designed for date-time values. If you attempt to convert date-time strings that are not formatted correctly, you may encounter issues. For instance, if the "Dates" column contains dates in the format YYYY-DD-MM, using astype() will result in an error.
df = pd.DataFrame({"Dates": ["2022-25-12", "2021-01-12", "2022-30-08"]})
df["NewDate_using_astype()"] = df["Dates"].astype("datetime64[ns]")
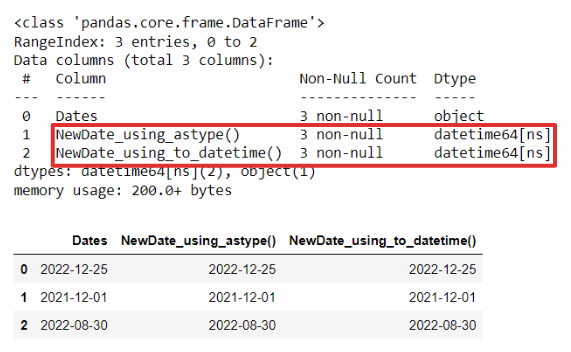
If you try to convert incorrectly formatted dates, astype() will throw a ValueError, as it expects the input in the YYYY-MM-DD format. Conversely, to_datetime() allows you to specify the input format through an optional parameter:
df["NewDate_using_to_datetime()"] = pd.to_datetime(df["Dates"], format='%Y-%d-%m')
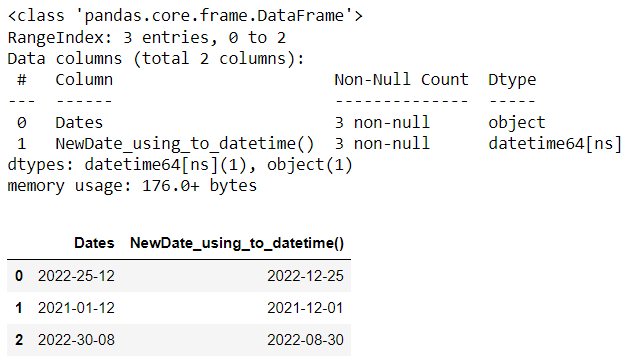
This flexibility allows to_datetime() to handle various date formats without errors.
Chapter 2: Error Management
Error handling is another critical aspect where these two methods diverge. Although to_datetime() may be slower, it excels in managing conversion errors.
Suppose your data contains invalid date strings, such as missing days or containing unexpected characters. For example:
df1 = pd.DataFrame({"Dates": ["2022-12-25", "2021-12-20", "2022-12-b", "2023-07-15", "2020- -31"]})
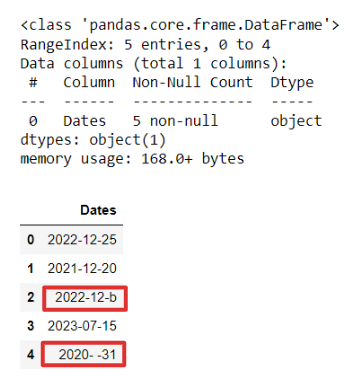
When using astype() to convert these values, you may encounter errors, as shown below:
df1["Dates"] = df1["Dates"].astype("datetime64[ns]")
Both methods will raise a TypeError and a ParserError by default. To avoid this, you can use the errors parameter with either method, setting it to "ignore" to bypass errors:
df1["Dates-astype-ignore"] = df1["Dates"].astype("datetime64[ns]", errors='ignore')
df1["Dates-to_datetime-ignore"] = pd.to_datetime(df1["Dates"], errors='ignore')
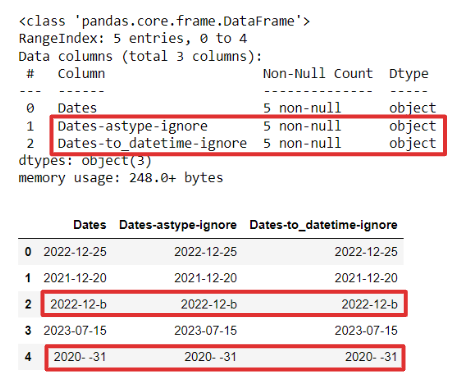
Ignoring errors retains the original input as strings, which does not help identify invalid entries. In this case, to_datetime() can use the coerce option, which converts valid date strings to datetime64[ns], while setting invalid ones to NaT (Not-a-Time):
df1["Dates-to_datetime-coerce"] = pd.to_datetime(df1["Dates"], errors='coerce')
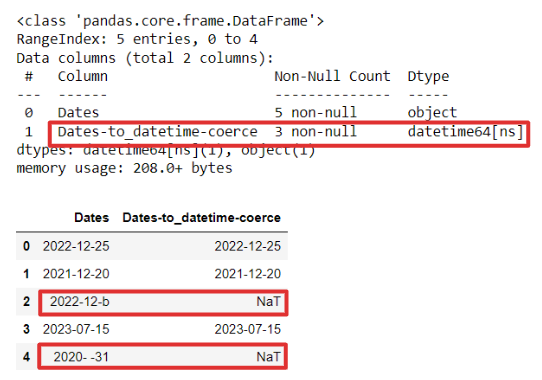
This approach not only converts valid entries but also highlights invalid ones, allowing for easier debugging.
In conclusion, while astype() offers speed and the ability to convert multiple columns at once, to_datetime() is generally the better choice for time-series data due to its handling of various date formats and error management.
If you're keen on enhancing your data science skills, consider becoming a Medium member for unlimited access to insightful articles. Also, sign up for my email list to stay updated on data science tips and tutorials.
Thank you for reading! Here are additional resources to enhance your proficiency with date-time data in Python and Pandas.
3 Powerful Tricks To Work With Date-Time Data In Python
Learn how to work with date and time data in Python using the datetime module.
towardsdatascience.com
3 Useful Pandas Tips To Work With Datetime Data
Quickly learn how to transform time series data in Python Pandas.
towardsdatascience.com
Chapter 3: Practical Videos
This video explores practical techniques for solving real-world data science tasks using Python's Pandas library.
In this video, learn how to change the data types of columns effectively in Pandas.