Mastering Object Prototypes in JavaScript: A Beginner's Guide
Written on
Chapter 1: Introduction to Object Prototypes
JavaScript, known for its flexibility and extensive use, fundamentally relies on objects. A solid understanding of object prototypes is vital for developers aiming to write code that is both efficient and easy to maintain. This article explores the core principles of object prototypes in JavaScript, offering clear explanations alongside practical coding examples to enhance your understanding of this key concept.
What Are Object Prototypes?
In JavaScript, each object possesses a prototype. Think of a prototype as a template that outlines the properties and methods an object can inherit. When an object is created in JavaScript, it automatically adopts properties and methods from its prototype. This mechanism of inheritance promotes code reusability and aids in the effective organization and structuring of your code.
Creating Objects with Prototypes
To begin, let’s look at how to create a basic object using a constructor function while establishing its prototype:
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.greet = function() {
return Hello, my name is ${this.name} and I am ${this.age} years old.;
};
const john = new Person('John', 30);
console.log(john.greet());
In the example above, we define a Person constructor function that accepts name and age as parameters. We then attach a greet method to the Person prototype, enabling instances of Person to introduce themselves.
Prototypal Inheritance
A significant aspect of object prototypes in JavaScript is the concept of prototypal inheritance. This feature allows an object to inherit properties and methods from its prototype. Let’s examine this with a further example:
function Student(name, age, grade) {
Person.call(this, name, age);
this.grade = grade;
}
Student.prototype = Object.create(Person.prototype);
Student.prototype.constructor = Student;
Student.prototype.displayInfo = function() {
return ${this.name} is ${this.age} years old and is in grade ${this.grade}.;
};
const alice = new Student('Alice', 25, 'A');
console.log(alice.displayInfo());
Here, we create a Student constructor function that leverages the Person constructor using Object.create. We then introduce a displayInfo method to the Student prototype, which provides details about the student.
The Prototype Chain
In JavaScript, objects can have prototypes that may themselves have further prototypes, forming a structure known as the prototype chain. This chain enables objects to inherit properties and methods from multiple levels up the hierarchy. Here’s an illustration:
function Teacher(name, age, subject) {
Person.call(this, name, age);
this.subject = subject;
}
Teacher.prototype = Object.create(Person.prototype);
Teacher.prototype.constructor = Teacher;
Teacher.prototype.teach = function() {
return ${this.name} teaches ${this.subject}.;
};
const bob = new Teacher('Bob', 40, 'Math');
console.log(bob.teach());
console.log(bob.greet());
In this case, we develop a Teacher constructor function that inherits from the Person constructor. The prototype chain for Teacher includes properties from both Person and Object prototypes.
Conclusion
Grasping the concept of object prototypes in JavaScript is crucial for mastering the language and crafting efficient code. By understanding how prototypes function and how objects inherit properties and methods, you can enhance your skills as a JavaScript developer.
Feel free to experiment with creating objects using prototypes, diving into prototypal inheritance, and exploring the prototype chain to enrich your comprehension of JavaScript's object-oriented aspects.
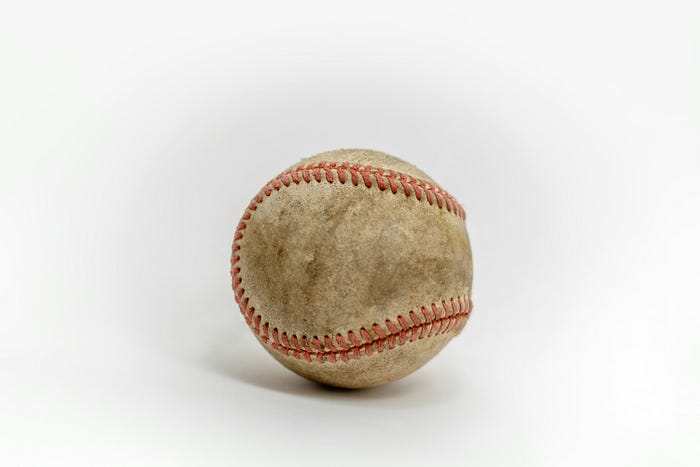
Chapter 2: Video Resources for Learning Prototypes
This video, titled "Prototypes In JavaScript & Object Creation Patterns: Simply Explained," offers an accessible overview of prototypes and their significance in JavaScript.
In the second video, "9.19: Prototypes in Javascript - p5.js Tutorial," viewers can explore practical applications of prototypes in a p5.js context.