Mastering JavaScript Basics: Your Comprehensive Guide for Interviews
Written on
Chapter 1: Introduction to the Fetch API
In the journey of mastering JavaScript, understanding the Fetch API is crucial. This guide covers the essentials you need for successful interview preparation, focusing on making GET requests.
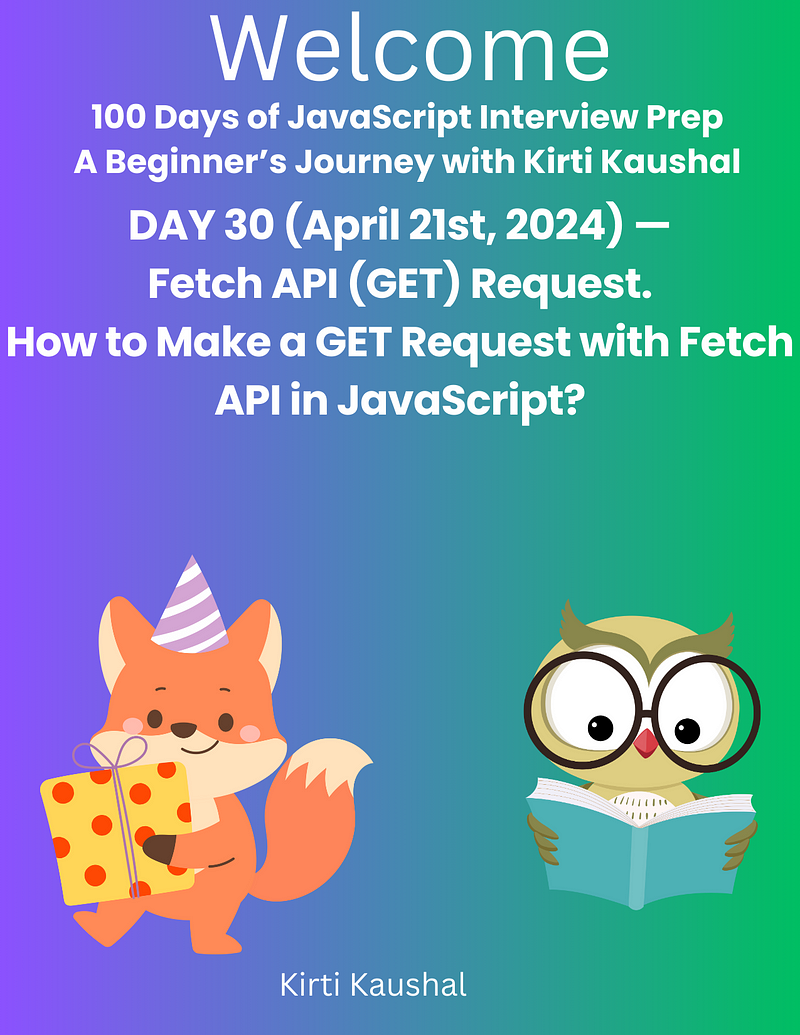
DAY 30 (April 21st, 2024) — Fetch API (GET) Request: A Practical Guide
As we reach Day 30 of our 100-day JavaScript challenge, we will explore how to perform a GET request using the Fetch API. This is an important skill when interacting with external APIs to retrieve data.
To illustrate this, we'll be utilizing JSONPlaceholder, a free and reliable fake API designed for testing and prototyping.
JSONPlaceholder JSONPlaceholder is supported by JSON Server and LowDB, managing around 3 billion requests each month. You can access it at:
Getting Started with Fetch API
Tip: The default method in the Fetch API is always GET.
Key Parameters of the Fetch API
Here are the main parameters you will work with:
- Method: This defines the type of request, such as "GET" or "POST", with GET being the default.
- Resource: This is the URL of the resource you wish to fetch, which can be a string or a Request object.
- Options (optional): An object for custom settings, including body, cache, headers, etc.
- Return Value: A Promise that resolves to a Response object.
- Exceptions: Handle network errors and syntax errors.
Basic Syntax
The syntax for using Fetch API is straightforward:
fetch(resource); // Basic resource fetch fetch(resource, options); // Fetch with options
For detailed information about the Fetch API, refer to the following resources:
Step-by-Step Example
#### Step 1: Fetching Data
To fetch data from our endpoint, use the following code:
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');}
return response.json(); // Parse the JSON data
})
.then(data => {
console.log(data);})
.catch(error => {
console.error('Fetch error:', error);});
You can test this code in a live JavaScript playground:
JavaScript Playground
#### Step 2: Network Monitoring
Open your browser's DevTools and navigate to the Network tab to observe the request and its parameters in real time.
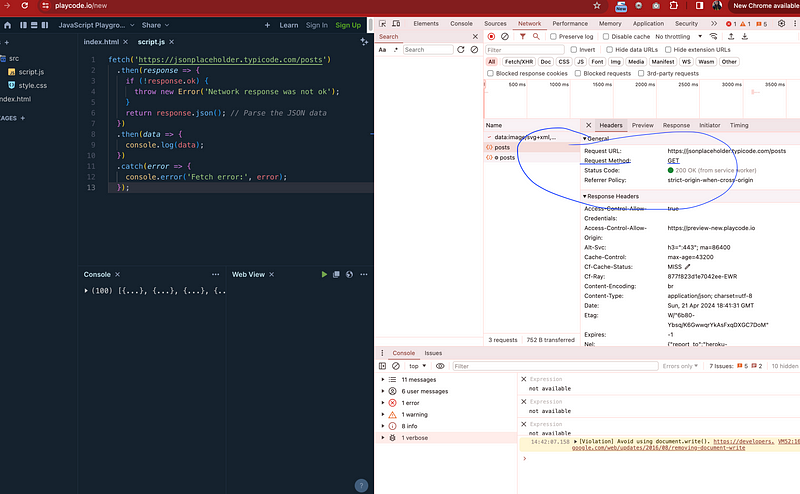
Handling Successful Responses
When working with the Fetch API, it's important to manage the promise returned. Here’s how you can handle successful responses:
- The Fetch API returns a promise.
- Use the then() method to process the resolved data.
- API responses may not always be in a usable format, so parsing is necessary.
Here’s a complete example for clarity:
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');}
return response.json(); // Convert to JSON
})
.then(data => {
console.log(data); // Log the JSON data})
.catch(error => {
console.error('Fetch error:', error); // Handle errors});
Error Handling in Fetch API
Understanding how to handle failures is essential when using the Fetch API. Consider these scenarios:
- Network Errors: These occur when there’s a loss of internet connection or server issues, causing the promise to reject.
- HTTP Errors: Even with a successful network request, the server may return an error status (e.g., 404 or 500).
Example of error handling:
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');}
return response.json(); // Parse JSON
})
.then(data => {
console.log(data); // Process the data})
.catch(error => {
console.error('Fetch error:', error); // Handle exceptions});
Conclusion
As we conclude our exploration of the Fetch API, remember that mastering these concepts is key for effective JavaScript development.
Tomorrow, we will delve deeper into the Fetch API with POST method examples.
Happy Coding and Stay Tuned! Kindness is essential — share knowledge and treat others with respect!
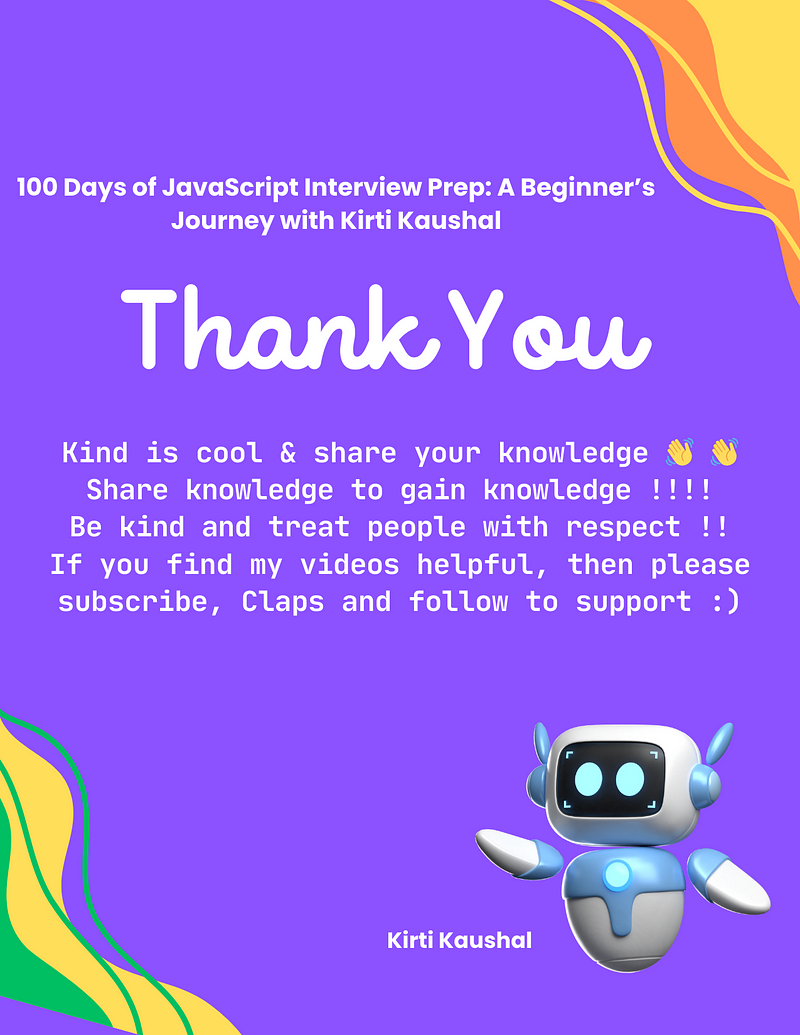
Chapter 2: Video Resources
For further learning, check out these insightful YouTube videos:
Video Title: Launching JavaScript Mastery Course | Advanced JavaScript in 30 Days Description: This video guides you through advanced JavaScript concepts in a structured 30-day course format.
Video Title: Mastering JavaScript in 30 Days! | LeetCode Challenge | NareshIT Description: Join this LeetCode challenge to sharpen your JavaScript skills, focusing on practical problem-solving techniques.