Unlocking the Power of Functional Programming in JavaScript
Written on
Understanding Functional Programming
Functional programming (FP) is a widely-adopted programming paradigm within JavaScript. This approach regards functions as first-class entities, making it straightforward to implement functional programming concepts in JavaScript.
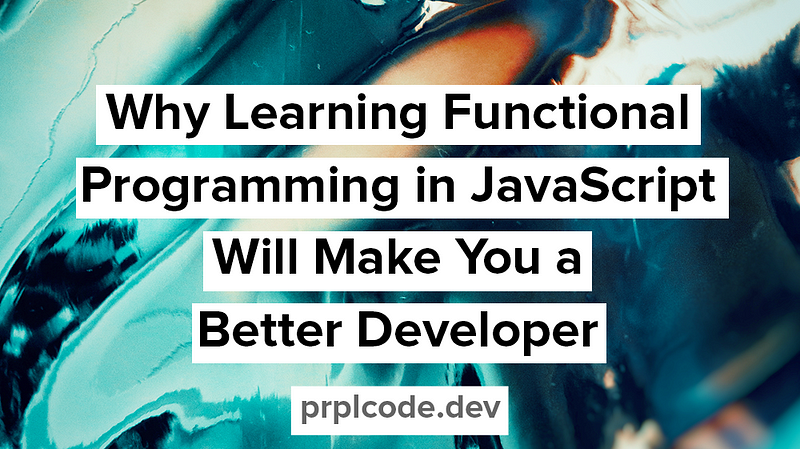
This article will introduce the foundational concepts of functional programming, its benefits, and how you can apply these principles in JavaScript.
What is Functional Programming?
Functional programming refers to a methodology for developing software based on specific guiding principles. The goal of these principles is to:
- Simplify the writing, testing, and debugging processes
- Enhance code readability and maintainability
- Boost overall developer productivity
Core Principles of Functional Programming
Pure Functions
Pure functions are a cornerstone of functional programming. They consistently yield the same output for identical inputs and do not produce side effects that alter the output. Side effects can include any form of interaction outside the function’s scope, such as reading from a database or using console logging.
Here’s a straightforward illustration of a pure function:
function add(a, b) {
return a + b;}
Handling I/O Operations
While applications often require input/output (I/O) operations, functional programming does not advocate for their removal. Instead, it emphasizes the separation of business logic from I/O processes. Side effects should be managed at the boundaries of processes, allowing for pure, testable business logic to exist in the core of the application.
JavaScript is not strictly a functional programming language; however, it allows for flexibility in how functions are implemented. In contrast, Haskell is a purely functional programming language that strictly enforces these principles.
Immutable State
In functional programming, the principle of immutable state dictates that states should remain unchanged. Rather than altering the state, it is more effective to create a copy of it. This may seem unconventional, but it offers greater safety, particularly in JavaScript where values can be passed by reference.
Here’s a simple example demonstrating this concept:
const originalState = { count: 1 };
const newState = { ...originalState, count: 2 };
Recursion
Functional programming avoids mutating state by using recursion instead of traditional loops like for or while. Functions such as map(), filter(), and reduce() are employed to iterate over lists.
Initially, I found the concept of recursion daunting. However, in JavaScript, these functions can greatly enhance readability and efficiency.
Let’s examine an example of using recursion:
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(n => n * 2);
Functional Programming Methods in JavaScript
As mentioned earlier, the map() function is a great starting point. It processes each element in a list and allows you to replace it with a new value. This function returns a new list while leaving the original list unchanged, adhering to functional programming principles.
In addition to map(), two other essential functions are filter() and reduce().
- The filter() function allows you to remove elements that do not meet specific criteria from a list.
const fruits = [{ name: 'apple', price: 4 }, { name: 'banana', price: 6 }];
const affordableFruits = fruits.filter(fruit => fruit.price <= 5);
- The reduce() function condenses a list of elements into a single value, which is particularly useful for numerical computations.
const total = [1, 2, 3, 4].reduce((acc, val) => acc + val, 0);
This function can be challenging to grasp, so I suggest checking out the MDN Web Docs for further clarification.
Conclusion
Now that you have a foundational understanding of functional programming and its advantages in JavaScript, you can begin to integrate these concepts into your coding practices. Although it may take some time to adapt, the benefits are significant. The functions we've explored are not exclusive to JavaScript but are prevalent across many programming languages.
Connect with me on Twitter, LinkedIn, or GitHub for more insights!
Chapter 2: Learning Resources
To deepen your understanding, here are some valuable resources:
This first video, "Learn Functional Programming in JavaScript," provides an overview of FP concepts and their applications in JavaScript.
The second video, "How I Learned JavaScript Functional Programming," shares personal insights and strategies for mastering FP.